python使用mediapiple+opencv识别视频人脸的实现
作者:拼命_小李 时间:2023-06-09 08:10:17
1、安装
pip install mediapipe
2、代码实现
# -*- coding: utf-8 -*-
"""
@Time : 2022/3/18 14:43
@Author : liwei
@Description:
"""
import cv2
import mediapipe as mp
mp_drawing = mp.solutions.drawing_utils
mp_face_mesh = mp.solutions.face_mesh
mp_face_detection = mp.solutions.face_detection
# 绘制人脸画像的点和线的大小粗细及颜色(默认为白色)
drawing_spec = mp_drawing.DrawingSpec(thickness=1, circle_radius=1)
cap = cv2.VideoCapture("E:\\video\\test\\test.mp4")# , cv2.CAP_DSHOW
# For webcam input:
# cap = cv2.VideoCapture(0)
with mp_face_detection.FaceDetection(
model_selection=0, min_detection_confidence=0.5) as face_detection:
while cap.isOpened():
success, image = cap.read()
if not success:
print("Ignoring empty camera frame.")
# If loading a video, use 'break' instead of 'continue'.
break
# To improve performance, optionally mark the image as not writeable to
# pass by reference.
image.flags.writeable = False
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
results = face_detection.process(image)
# Draw the face detection annotations on the image.
image.flags.writeable = True
image = cv2.cvtColor(image, cv2.COLOR_RGB2BGR)
if results.detections:
box = results.detections[0].location_data.relative_bounding_box
xmin = box.xmin
ymin = box.ymin
width = box.width
height = box.height
xmax = box.xmin + width
ymax = ymin + height
cv2.rectangle(image, (int(xmin * image.shape[1]),int(ymin* image.shape[0])), (int(xmax* image.shape[1]), int(ymax* image.shape[0])), (0, 0, 255), 2)
# for detection in results.detections:
# mp_drawing.draw_detection(image, detection)
# Flip the image horizontally for a selfie-view display.
cv2.imshow('MediaPipe Face Detection', cv2.flip(image, 1))
if cv2.waitKey(5) & 0xFF == 27:
break
cap.release()
效果
3、更新 mediapiple+threadpool+opencv实现图片人脸采集效率高于dlib
# -*- coding: utf-8 -*-
"""
@Time : 2022/3/23 13:43
@Author : liwei
@Description:
"""
import cv2 as cv
import mediapipe as mp
import os
import threadpool
mp_drawing = mp.solutions.drawing_utils
mp_face_mesh = mp.solutions.face_mesh
mp_face_detection = mp.solutions.face_detection
savePath = "E:\\saveImg\\"
basePath = "E:\\img\\clear\\20220301\\"
def cut_face_img(file):
# print(basePath + file)
img = cv.imread(basePath + file)
with mp_face_detection.FaceDetection(
model_selection=0, min_detection_confidence=0.5) as face_detection:
img.flags.writeable = False
image = cv.cvtColor(img, cv.COLOR_RGB2BGR)
results = face_detection.process(image)
image = cv.cvtColor(image, cv.COLOR_RGB2BGR)
image.flags.writeable = True
if results.detections:
box = results.detections[0].location_data.relative_bounding_box
xmin = box.xmin
ymin = box.ymin
width = box.width
height = box.height
xmax = box.xmin + width
ymax = ymin + height
x1, x2, y1, y2 = int(xmax * image.shape[1]), int(xmin * image.shape[1]), int(
ymax * image.shape[0]), int(ymin * image.shape[0])
cropped = image[y2:y1, x2:x1]
if cropped.shape[1] > 200:
cv.imwrite(savePath + file, cropped)
print(savePath + file)
if __name__ == '__main__':
data = os.listdir(basePath)
pool = threadpool.ThreadPool(3)
requests = threadpool.makeRequests(cut_face_img, data)
[pool.putRequest(req) for req in requests]
pool.wait()
来源:https://blog.csdn.net/m0_43432638/article/details/123684319
标签:opencv,识别,视频人脸


猜你喜欢
python实现银行账户系统
2023-05-27 17:49:08
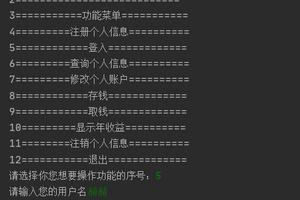
JS比较两个数值的大小实例
2024-04-17 10:41:03
Pandas.DataFrame时间序列数据处理的实现
2022-09-20 08:43:41
python tornado开启多进程的几种方法
2021-09-18 22:28:17
ES6入门教程之let和const命令详解
2024-05-22 10:37:07
python3中join和格式化的用法小结
2022-03-02 01:45:22
python获取时间及时间格式转换问题实例代码详解
2021-12-20 12:46:20
关于ORA-04091异常的出现原因分析及解决方案
2024-01-22 22:29:20
phpmyadmin 4+ 访问慢的解决方法
2024-05-05 09:31:28
Python 获取命令行参数内容及参数个数的实例
2023-11-03 18:00:11
python机器学习sklearn实现识别数字
2023-08-18 17:57:19

python 循环while和for in简单实例
2021-12-11 03:16:48
Vue网页html转换PDF(最低兼容ie10)的思路详解
2024-06-07 15:25:12
python使用wxPython打开并播放wav文件的方法
2023-08-23 10:30:58
Python技法之如何用re模块实现简易tokenizer
2021-08-04 17:03:52
js动态显示当前日期,时间和星期代码
2007-08-14 12:31:00
Python RuntimeError: thread.__init__() not called解决方法
2022-12-22 17:11:46
python list 查询是否存在并且并返回下标的操作
2023-06-20 12:05:43

常用的数据库访问方式是什么?
2009-11-01 15:08:00
Python 如何强制限定小数点位数
2022-10-01 15:25:43