python批量修改文件名的实现代码
作者:mdxy-dxy 时间:2023-08-02 19:06:53
#coding:utf-8
#批量修改文件名
import os import re import datetime
re_st = r'(\d+)\+\s?\((\d+)\)'
#用于匹配旧的文件名,需含分组 re_match_old_file_name = re.compile(re_st)
#要修改的目录 WORKING_PATH = r'F:\Gallery'
#----------------------------------------------------------------------
def rename_fomat(name):
"""
文件重命名格式组织模块(一般修改这里就可以了)
NOTE:返回类型必须是unicode
"""
if name:
re_rn = re_match_old_file_name.findall(name)
if re_rn and re_rn != []:
re_rn = re_rn[0]
num = int(re_rn)
new_nm = u'NO.%04d' % ( num)
return new_nm
#----------------------------------------------------------------------
def logs(error):
"""
错误记录
"""
log = ''
LOG_FILE = open(r'./log.txt', 'a')
live_info ="""
==========
Time : %s
title : %s
Path :
%s
==========
""" % (
datetime.datetime.now(),
str(error['title']),
str(error['index']),
)
log += live_info
errors = error['error_paths']
for item in errors:
item = '%s\n' % item
log += item
log = log.encode('utf-8')
try:
LOG_FILE.write(log)
except IOError:
print u'写入日志失败'
finally:
LOG_FILE.close()
#----------------------------------------------------------------------
def rename(old, new):
"""
文件重命名模块
return:
0:rename success
1:the new path is exists
-1:rename failed
"""
if not os.path.exists(new):
try:
os.renames(old, new)
return 0
except IOError:
print 'path error:', new
return -1
else:
return 1
#----------------------------------------------------------------------
def get_dirs(path):
"""
获取目录列表
"""
if os.path.exists(path):
return os.listdir(path)
else:
return -1
#----------------------------------------------------------------------
def get_input_result(word, choice):
"""
获取正确的输入结果
"""
correct_result = set(choice)
word = '===%s?\n[in]:' % (word)
while True:
in_choice = raw_input(word)
if in_choice in correct_result: return in_choice
#----------------------------------------------------------------------
def batch_rename(index, dirs = []):
"""
批量修改文件
"""
index = unicode(index)
errors = []
if dirs == []:
dirs = get_dirs(path = index)
if dirs and dirs != []:
for item in dirs:
item = unicode(item)
new_name = rename_fomat(item)
if new_name :
old_pt = u'%s\\%s'% (index, item)
new_pt = u'%s\\%s'% (index, new_name)
res_rn = rename(old_pt, new_pt)
if res_rn != 0:
errors.append(item)
else:
errors.append(item)
if errors and errors != []:
print 'Rename Failed:'
logs({
'index': index,
'title': 'Rename Failed' ,
'error_paths': errors,
})
for i, item in enumerate(errors):
print item, '|',
if i % 5 == 4:
print ''
print ''
else:
return -1
#----------------------------------------------------------------------
def batch_rename_test(index):
"""
测试
返回过滤结果
"""
index = unicode(index)
errors = []
correct = []
dirs = get_dirs(path = index)
if dirs and dirs != []:
for x, item in enumerate(dirs):
item = unicode(item)
new_name = rename_fomat(item)
if new_name :
correct.append(item)
old_pt = u'%s\\%s'% (index, item)
new_pt = u'%s\\%s'% (index, new_name)
print '[%d]O: %s' % ( x + 1, old_pt)
print '[%d]N: %s' % ( x + 1, new_pt)
else:
errors.append(item)
if errors and errors != []:
print 'Not Match:'
logs({
'index': index,
'title': 'Not Match',
'error_paths': errors,
})
for i, item in enumerate(errors):
print item, '|',
if i % 5 == 4:
print ''
print ''
return correct
#----------------------------------------------------------------------
def manage(index):
"""
程序组织块
"""
file_filter = batch_rename_test(index)
do_choice = get_input_result(
word = 'Do with this(y / n)',
choice = ['y', 'n']
)
if do_choice == 'y':
batch_rename(index, dirs= file_filter)
print 'Finished !'
if __name__ == '__main__':
path = WORKING_PATH
manage(index = path)
标签:python,批量修改,文件名


猜你喜欢
深入了解Python在HDA中的应用
2023-08-02 06:59:47

基于python进行桶排序与基数排序的总结
2023-06-13 17:32:33
Windows系统彻底卸载SQL Server通用方法(推荐!)
2024-01-17 19:03:25

python 的生产者和消费者模式
2021-09-18 07:19:45

NopCommerce架构分析之(八)多语言支持
2024-05-13 09:15:53
30步检查SQL Server安全列表
2008-12-18 14:28:00
基于python-pptx库中文文档及使用详解
2023-11-30 12:06:13

python3+selenium获取页面加载的所有静态资源文件链接操作
2022-11-02 19:18:36

vue实现验证码倒计时按钮
2024-04-09 10:49:05

Python打开文件,将list、numpy数组内容写入txt文件中的方法
2023-12-16 21:23:17
SQL SERVER 中构建执行动态SQL语句的方法
2024-01-22 10:01:46
Javascript优化(文件瘦身)
2008-06-02 13:20:00
python因子分析的实例
2021-12-29 18:20:44

Go语言Mock使用基本指南详解
2024-05-08 10:15:03
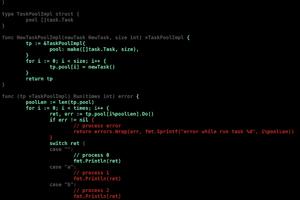
Python中摘要算法MD5,SHA1简介及应用实例代码
2023-12-12 00:36:41
python私有属性和方法实例分析
2023-11-21 06:16:13
详解django+django-celery+celery的整合实战
2022-11-14 12:25:13

Vue.js鼠标悬浮更换图片功能
2024-04-30 10:42:35

基于python分享极坐标下的几类典型曲线
2023-05-02 18:18:37

MSSQL MySQL 数据库分页(存储过程)
2012-01-29 18:30:20